Image Generation
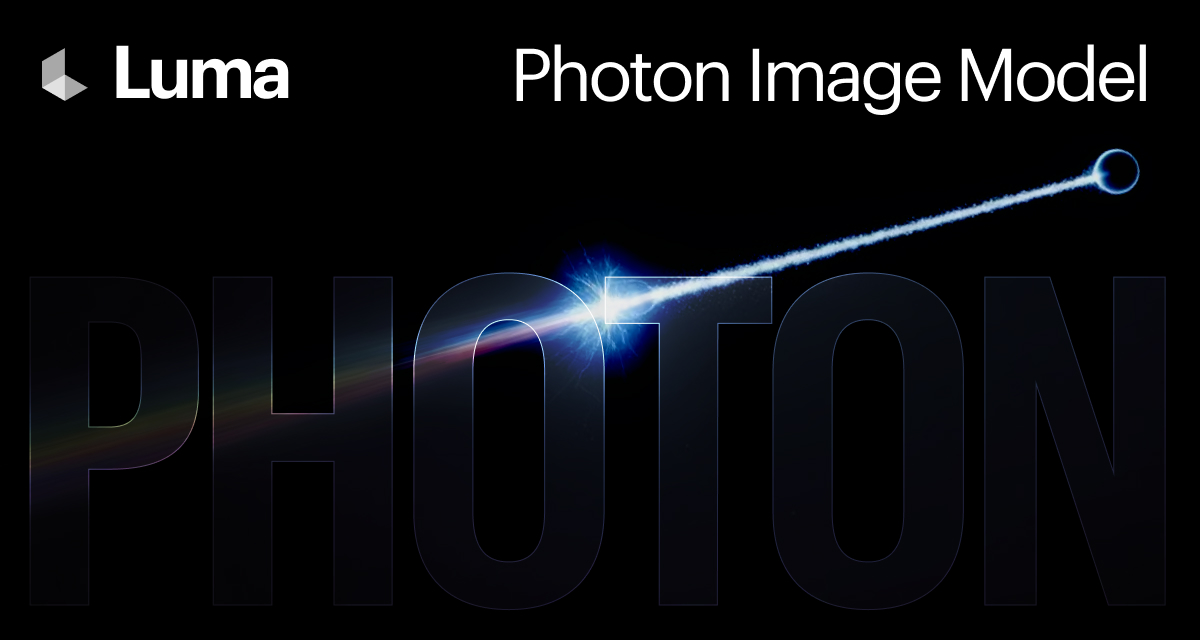
Installation
npm install lumaai
https://www.npmjs.com/package/lumaai
Authentication
- Get a key from https://lumalabs.ai/dream-machine/api/keys
In JavaScript, you can pass the API key using the authToken
parameter when creating the client
const { LumaAI } = require('lumaai');
const client = new LumaAI({
authToken: process.env.LUMAAI_API_KEY
});
Polling for Generation Status and Retrieving Image
- Right now the only supported way is via polling
- The
create
endpoint returns an id which is an UUID V4 - You can use it to poll for updates (you can see the image at
generation.assets.image
)
Usage Example
npm install node-fetch
const fetch = require('node-fetch');
const fs = require('fs');
const { LumaAI } = require('lumaai');
const client = new LumaAI({ authToken: process.env.LUMAAI_API_KEY });
async function generateImage() {
let generation = await client.generations.image.create({
prompt: "A teddy bear in sunglasses playing electric guitar and dancing"
});
let completed = false;
while (!completed) {
generation = await client.generations.get(generation.id);
if (generation.state === "completed") {
completed = true;
} else if (generation.state === "failed") {
throw new Error(`Generation failed: ${generation.failure_reason}`);
} else {
console.log("Dreaming...");
await new Promise(r => setTimeout(r, 3000)); // Wait for 3 seconds
}
}
const imageUrl = generation.assets.image;
const response = await fetch(imageUrl);
const fileStream = fs.createWriteStream(`${generation.id}.jpg`);
await new Promise((resolve, reject) => {
response.body.pipe(fileStream);
response.body.on('error', reject);
fileStream.on('finish', resolve);
});
console.log(`File downloaded as ${generation.id}.jpg`);
}
generateImage();
Aspect Ratio and Model
For all your requests, you can specify the aspect ratio you want and also the model to be used.
Aspect ratio
You can choose between the following aspect ratios:
- 1:1
- 3:4
- 4:3
- 9:16
- 16:9 (default)
- 9:21
- 21:9
To use it, simply include a new key under your payload:
prompt: "A teddy bear in sunglasses playing electric guitar and dancing",
aspect_ratio: "3:4"
Model
You can choose from our two model versions:
- photon-1 (default)
- photon-flash-1
To use it, simply include a new key under your payload:
prompt: "A teddy bear in sunglasses playing electric guitar and dancing",
model: "photon-flash-1"
Text to Image
const generation = await client.generations.image.create({
prompt: "A teddy bear in sunglasses playing electric guitar and dancing"
});
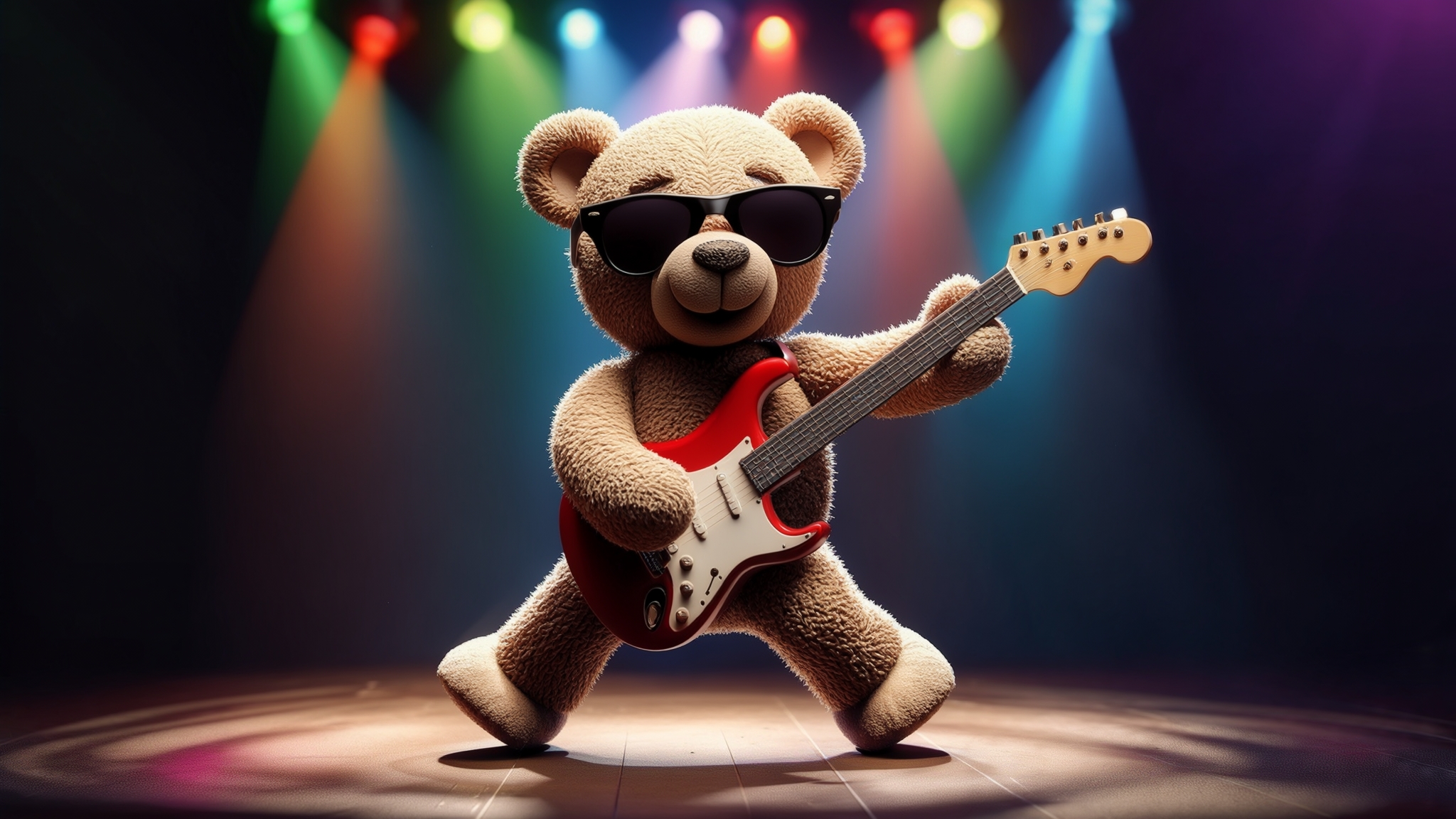
With aspect ratio and model
const generation = await client.generations.image.create({
prompt: "A teddy bear in sunglasses playing electric guitar and dancing",
aspect_ratio: "3:4",
model: "photon-1"
});
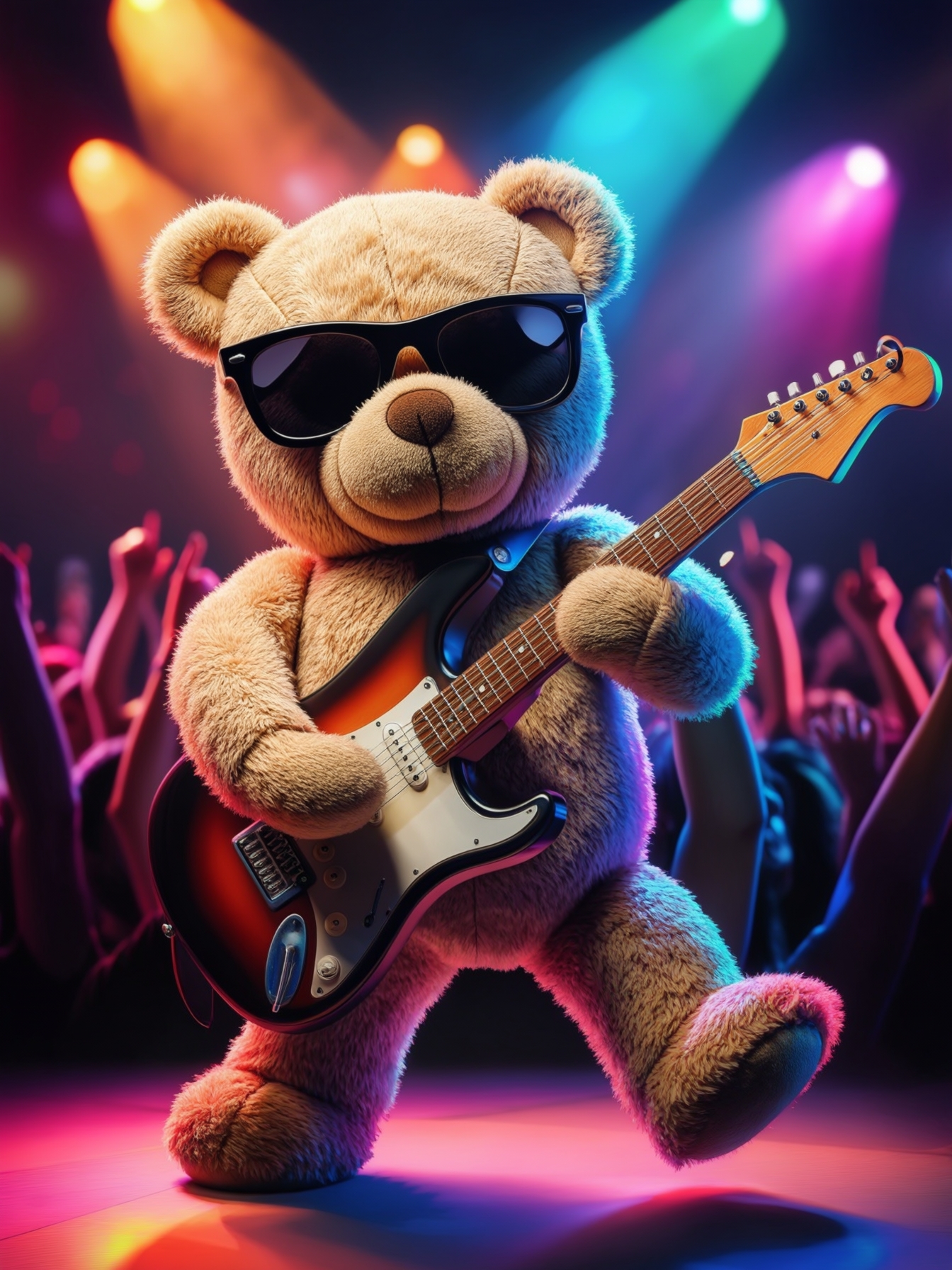
Image Reference
Image URL
You should upload and use your own cdn image urls, currently this is the only way to pass an image
This feature allows you to guide your generation using a combination between images and prompt. You can use up to 4 images as references. This feature is very useful when you want to create variations of an image or when you have a concept that is hard to describe, but easy to visualize. You can use the weight
key to tune the influence of the images.
const generation = await client.generations.image.create({
prompt: "sunglasses",
image_ref: [
{
"url": "https://storage.cdn-luma.com/dream_machine/7e4fe07f-1dfd-4921-bc97-4bcf5adea39a/video_0_thumb.jpg",
"weight": 0.85
}
]
});
Style Reference
Image URL
You should upload and use your own cdn image urls, currently this is the only way to pass an image
As the name suggests, this feature is used when you want to apply an specific style to your generation. You can use the weight
key to tune the influence of the style image reference.
const generation = await client.generations.image.create({
prompt: "dog",
style_ref: [
{
"url": "https://staging.storage.cdn-luma.com/dream_machine/400460d3-cc24-47ae-a015-d4d1c6296aba/38cc78d7-95aa-4e6e-b1ac-4123ce24725e_image0c73fa8a463114bf89e30892a301c532e.jpg",
"weight": 0.8
}
]
});
Character Reference
Image URL
You should upload and use your own cdn image urls, currently this is the only way to pass an image
Character Reference is a feature that allows you to create consistent and personalized characters. Below, you can see how to use it. One thing important to say is that you can use up to 4 images of the same person to build one identity. More images, better the character representation will be.
const generation = await client.generations.image.create({
prompt: "man as a warrior",
character_ref: {
"identity0": {
"images": [
"https://staging.storage.cdn-luma.com/dream_machine/400460d3-cc24-47ae-a015-d4d1c6296aba/38cc78d7-95aa-4e6e-b1ac-4123ce24725e_image0c73fa8a463114bf89e30892a301c532e.jpg"
]
}
}
});
Modify Image
Image URL
You should upload and use your own cdn image urls, currently this is the only way to pass an image
Changing colors of images
This feature works really well to change objects, shapes, etc. But when it comes to changing colors, it is harder to get it right. One recommendation is to use a lower
weight
value, something between 0.0 and 0.1.
Modify feature allows you to refine your images by simply prompting what change you want to make. You can use the weight
key to specify the influence of the input image. Higher the weight, closer to the input image but less diverse (and creative).
const generation = await client.generations.image.create({
prompt: "transform all the flowers to sunflowers",
modify_image_ref: {
"url": "https://staging.storage.cdn-luma.com/dream_machine/400460d3-cc24-47ae-a015-d4d1c6296aba/38cc78d7-95aa-4e6e-b1ac-4123ce24725e_image0c73fa8a463114bf89e30892a301c532e.jpg",
"weight": 1.0
}
});
Generations
Get generation with id
const generation = await client.generations.get("4fac0ef4-b336-45bf-a5dc-6de436cfbd62");
List all generations
const generation = await client.generations.list();
Delete generation
const generation = await client.generations.delete("4fac0ef4-b336-45bf-a5dc-6de436cfbd62");
Docs
https://www.npmjs.com/package/lumaai
Please see API docs for API reference.
How to get a callback when generation has an update
- It will get status updates (dreaming/completed/failed)
- It will also get the video url as part of it when completed
- It's a
POST
endpoint you can pass, and request body will have the generation object in it - It expected to be called multiple times for a status
- If the endpoint returns a status code other than 200, it will be retried max 3 times with 100ms delay and the request has a 5s timeout
example
const generation = await client.generations.create({
prompt: "A teddy bear in sunglasses playing electric guitar and dancing",
callback_url: "<your_api_endpoint_here>"
});
Updated 6 months ago